This article contains 11 Free Java Projects for Beginners [With Source Code]. Best Free Java Projects with source code on java, php, android, spring, hibernate, node.js, angularjs, c programming, html, css, javascript, jquery, ajax, xml, web etc. Online exam project in java will come in our next post. See some JApps (Java Application World) and Fee Reports from the similar articles.
What is Java?
Java is a general-purpose, object-oriented programming language that was developed and produced by John Gosling in 1995 at Sun Microsystems. It was created with the WORA principle in mind, which stands for “Write Once, Run Anywhere.” Compilated Java code, on the other hand, will run on any platform that supports Java without the need to recompile it.
Java has a wide range of applications in the field of mobile development, with web application development being one of the most common. It also has uses in desktop systems, Web servers and application servers, gaming, and database connections. It also provides assistance in the areas of integrated devices and technical applications.
You can find java projects with source code in this post. Because of the following applications, most developers use it for their projects:
- Simple
- Object-Oriented
- Portable
- Platform independent
- Secured
- Robust
- Architecture neutral
- Interpreted
- High Performance
- Multithreaded
- Distributed
- Dynamic.
To know more about Java, Checkout our related articles.
Java IDEs to Start Building Projects
IDEs | Online Editors |
MyEclipse | Codiva |
IntelliJ IDEA | JDoodle |
NetBeans | Rextester |
Dr. Java | Online GDB |
Blue J | Browxy |
JDeveloper | IDE One |
Java IDEs is a good place to start if you want to learn more about IDEs and editors.
Best Free and Simple Java Projects for Beginners
Check out these top Java Project Ideas to help you get started with Java programming and advance your career with these beginner-level Java projects.
1. Smart City Project
The Smart City project provides information about hotels, taxis, air ticket booking, retail specifics, and city news to tourists and other visitors in a city. As a result, it acts as a city guide for travellers. It is a Java-based web-based app that solves the majority of the problems that every new tourist faces while visiting a new location, such as pathfinding, hotel checking, ticket booking, and more.
Source Code: Smart City Project
2. Currency Converter
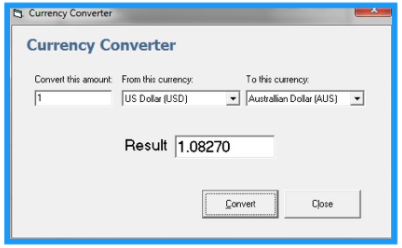
We all know that different nations have different currencies, and these currencies fluctuate in value on a regular basis. When transferring funds, people must be aware of the most recent currency exchange rate. As a result, the currency converter is a small Java project that offers a web-based GUI for exchanging/converting money between different currencies. It is built with Ajax, Java servlets, and other web features. Such applications have been used in the fields of industry, stocks, and finance.
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package com.exchange;
import java.io.*;
import java.net.*;
import java.util.*;
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.InputStream;
import java.net.*;
import com.google.gson.*;
/**
*
* @author pakallis
*/
class Recv
{
private String lhs;
private String rhs;
private String error;
private String icc;
public Recv(
{
}
public String getLhs()
{
return lhs;
}
public String getRhs()
{
return rhs;
}
}
public class Convert extends HttpServlet {
/**
* Processes requests for both HTTP <code>GET</code> and <code>POST</code> methods.
* @param request servlet request
* @param response servlet response
* @throws ServletException if a servlet-specific error occurs
* @throws IOException if an I/O error occurs
*/
protected void processRequest(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
String query = "";
String amount = "";
String curTo = "";
String curFrom = "";
String submit = "";
String res = "";
HttpSession session;
resp.setContentType("text/html;charset=UTF-8");
PrintWriter out = resp.getWriter();
/*Read request parameters*/
amount = req.getParameter("amount");
curTo = req.getParameter("to");
curFrom = req.getParameter("from");
/*Open a connection to google and read the result*/
try {
query = "http://www.google.com/ig/calculator?hl=en&q=" + amount + curFrom + "=?" + curTo;
URL url = new URL(query);
InputStreamReader stream = new InputStreamReader(url.openStream());
BufferedReader in = new BufferedReader(stream);
String str = "";
String temp = "";
while ((temp = in.readLine()) != null) {
str = str + temp;
}
/*Parse the result which is in json format*/
Gson gson = new Gson();
Recv st = gson.fromJson(str, Recv.class);
String rhs = st.getRhs();
rhs = rhs.replaceAll("�", "");
/*we do the check in order to print the additional word(millions,billions etc)*/
StringTokenizer strto = new StringTokenizer(rhs);
String nextToken;
out.write(strto.nextToken());
nextToken = strto.nextToken();
if( nextToken.equals("million") || nextToken.equals("billion") || nextToken.equals("trillion"))
{
out.println(" "+nextToken);
}
} catch (NumberFormatException e) {
out.println("The given amount is not a valid number");
}
}
// <editor-fold defaultstate="collapsed" desc="HttpServlet methods. Click on the + sign on the left to edit the code.">
/**
* Handles the HTTP <code>GET</code> method.
* @param request servlet request
* @param response servlet response
* @throws ServletException if a servlet-specific error occurs
* @throws IOException if an I/O error occurs
*/
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
processRequest(request, response);
}
/**
* Handles the HTTP <code>POST</code> method.
* @param request servlet request
* @param response servlet response
* @throws ServletException if a servlet-specific error occurs
* @throws IOException if an I/O error occurs
*/
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
processRequest(request, response);
}
/**
* Returns a short description of the servlet.
* @return a String containing servlet description
*/
@Override
public String getServletInfo() {
return "Short description";
}// </editor-fold>
}
3. Number Guessing Game
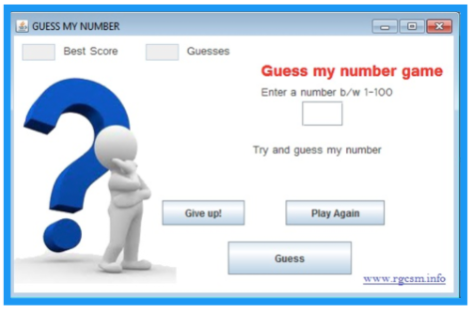
The fun and simple project “Guess the Number” is a short Java project that allows the user to guess the number created by the machine and includes the steps below:
- The method produces a random number between 1 and 100 from a given set.
- In a shown dialogue box, the user is asked to input their assigned phone number.
- The programme then determines whether the entered number corresponds to the guesses or is higher or lower than the generated number.
- The game begins with the player attempting to guess the number.
You may also provide additional information such as:
- Keeping the number of attempts to a minimum.
- More rounds are being added.
- The score is being shown.
- Points are awarded depending on the number of attempts.
Source Code
package guessinggame;
* Java game “Guess a Number” that allows user to guess a random number that has been generated.
*/
import javax.swing.*;
public class GuessingGame {
public static void main(String[] args) {
int computerNumber = (int) (Math.random()*100 + 1);
int userAnswer = 0;
System.out.println("The correct guess would be " + computerNumber);
int count = 1;
while (userAnswer != computerNumber)
{
String response = JOptionPane.showInputDialog(null,
"Enter a guess between 1 and 100", "Guessing Game", 3);
userAnswer = Integer.parseInt(response);
JOptionPane.showMessageDialog(null, ""+ determineGuess(userAnswer, computerNumber, count));
count++;
}
}
public static String determineGuess(int userAnswer, int computerNumber, int count){
if (userAnswer <=0 || userAnswer >100) {
return "Your guess is invalid";
}
else if (userAnswer == computerNumber ){
return "Correct!\nTotal Guesses: " + count;
}
else if (userAnswer > computerNumber) {
return "Your guess is too high, try again.\nTry Number: " + count;
}
else if (userAnswer < computerNumber) {
return "Your guess is too low, try again.\nTry Number: " + count;
}
else {
return "Your guess is incorrect\nTry Number: " + count;
}
}
}
4. Brick Breaker Game
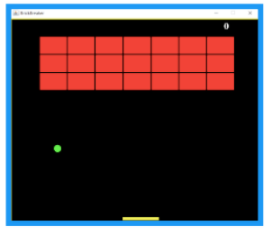
The bricks in the Brick Breaker game are aligned at the top of the frame. The player is portrayed by a small ball that is mounted at the bottom of the screen on a small platform. The platform on the computer can be switched from left to right using the arrow keys on the keyboard. The base is used by the player to keep the ball moving. The target is to smash as many bricks as possible while avoiding hitting the ball with your platform. The project makes extensive use of Java swing, OOPS principles, and other programming techniques.
Source Code: Brick Breaker Game
5. Data Visualization Software
Data visualisation is the process of creating a presentation and visualising data in a graphical or pictorial format. Since it is closely linked to information graphics and simulation, mathematical graphics, and science visualisation, data visualisation has been an active area of research and growth.
The project uses data visualisation to show node connectivity in networking. The mouse or trackpad may be used to transfer the node connectivity to various positions. The below are the project’s priorities and objectives:
- Knowledge is communicated effectively and clearly through graphical and pictorial means.
- It should be practical as well as attractive.
- It should be able to easily communicate ideas and have necessary insights into large amounts of data and knowledge.
When data is viewed or depicted as charts or graphs rather than report documents, data visualisation software makes it easier for the user to interpret and comprehend the details.
Source Code: Data Visualization Software
6. ATM Interface
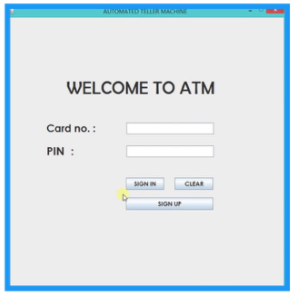
We’ve all seen ATMs in our cities, and they’re all Java-based. This multi-class project is a console-based programme with five distinct levels. The user is asked for their user id and pin when the device first starts up. After successfully entering the information, the ATM’s functions are unlocked. The project requires the following operations to be carried out:
- Transactions History
- Withdraw
- Deposit
- Transfer
- Quit
Source Code: ATM Interface
7. Web Server Management System
This webserver management system project, dubbed “E-Space,” is concerned with the content, maintenance, and management of the webserver. In today’s world of fast-moving e-Commerce websites, web servers are deemed worthy alternatives for businesses looking to make their goods accessible on the internet.
The project offers options for a company’s online activity by delivering server support for the company.
The below are the project’s goals:
- To determine if the user is an individual, a company, or just another web server.
- Determine the individual’s, company’s, or web server’s physical location.
- Consumers are aware of the protection and privacy policies.
- Determine the URL Authorities and URL Names.
- Maintain customer partnerships with the company’s online services.
Source Code: Web Server Management System
8. Airline Reservation System
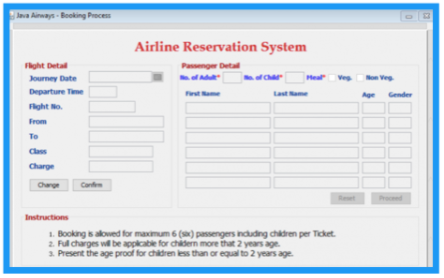
The software is web-based and has an open architecture. This ensures it can adapt to the changing demands of the airline industry by adding new systems and features. Internet transactions, fares, inventory, and e-ticket operations are all part of the initiative.
User authentication, password, reservation, and cancellation are the four main modules of the programme. The software makes connectivity over a TCP/IP network protocol, making internet and intranet communication more accessible across the world.
The below are the project’s key features:
- Ticket reservation and cancellation.
- Device operations are automated.
- Customer service is quick to respond.
- Execute account processing and routeing tasks.
- Keep track with all passengers.
Source Code: Airline Reservation System
9. Online Book Store
This initiative is mostly designed for bookstores and shops to computerise the book ordering process. This goes as the operating mechanisms of all stores are being digitised as technology advances. The project’s goal is to develop an effective and dependable online bookselling and purchasing network that will benefit both buyers and sellers. It saves time for buyers by allowing entry from anywhere and delivering home delivery.
The proposed web-based project also automatically tracks sold and stock books in the database.
The below are the project’s key features:
- The Database can only be used by active users.
- Books may be categorised by price, description, and other factors.
- The books that were purchased arrived safely.
- For both the store manager and the client, the project is quick and effective.
- The project must be dependable in order to conduct online transactions.
Source Code: Online Book Store
10. Snake Game in Java
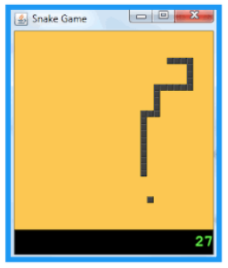
If you were a kid or an adult in the 1990s, you have most likely played this game on your tablet. Have you ever considered making this game? The aim of this game is to get the snake to consume the tokens that are its food without touching the screen’s boundary. Lastly, the score is changed every time the snake eats the token. When the snake reaches the boundary, the player loses and the final score is shown.
Source Code: Snake Game in Java
11. Email Application
The Email Application project is ideal if you’re still looking for more java coding experience. In fact, this project is more advanced than the previous one, but it is still a beginner’s project.
The best thing about this project is that you’ll be given requirements much as you are in a real-world project. You’ll also learn how to use OOPs principles like encapsulation to create a random password using the Math.random algorithm. You will be coding basic email operations such as setting the mailbox space. Additionally, you will be setting the alternative email, changing the password, and so on in this simple email programme.
Conclusion
That brings us to the Java projects that we encounter in our daily lives. Be it playing games as well as withdrawing money from ATMs. It can even be shopping online or even reserving an airline ticket Java code helps through all these tasks. Java is a stable and safe language, which is why it is the language of choice for developers working on such projects.
Learning to code in Java opens up a world of possibilities. So get ready and try these projects to improve your Java profile. You may also post a link to your GitHub attempt in the comments section below. Have you tried any other Java ventures that you enjoyed? Let us know and we’ll let the rest of the world know. Good luck.
Programming Resources and Articles You may like: Java Developer Testing Tools
- 11 Tools Every Java Developer Must Learn this Year
- 10 Best Programming Language to Learn as a Beginner
- Top 10 Reasons to Learn Python Programming Language Today
- Java Developer Testing Tools and Libraries programmers can use
- Web Developer RoadMap for Basic Skills, Frond End & Back End
- DevOps RoadMap & Courses to Becoming a Certified Engineer
- DevOps and Containers | Benefits, Metrics, Challenges & Risk Management
- 5 Best Spring Security Online Training Courses for Java Developers
- 10 Best AWS Certifications for IT Beginners & Professionals
- 10 Best Coursera Online Courses and Certifications for Tech Jobs